Rodrigues’ formula
In this post, I will introduce you to a great tool: Rodrigues’ formula. This is not the Rodrigues associated with Tarantino’s films, but you’ll soon see that he is just as brilliant. Let’s dive in!
I often encounter geometric problems in the projects I work on. Since these projects require runtime solutions, I perform analytical derivations and calculations on paper for optimization purposes. Many people struggle when dealing with rotation-related problems. In this post, I would like to present a very powerful tool: the operator representation of Rodrigues’ rotation formula. This formula enables elegant analytical proofs for rotation-related problems without the need to work with quaternions or matrices. If you’re a physicist, I believe you’ll particularly appreciate this approach and might even wonder, why wasn’t this covered in my studies?!
Rodrigues’ formula for a rotation $R$ by an angle $\theta$ around a direction $u$ is given by
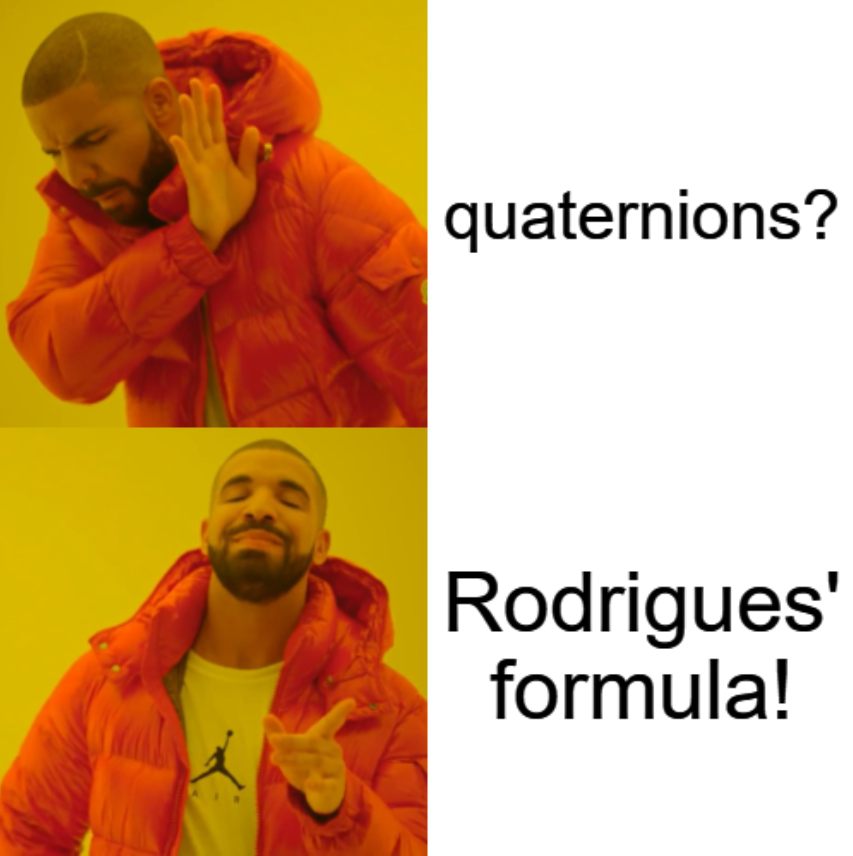
Example
Consider a sphere of radius $r$ at point $A$, a plane defined by point $B$ and normal direction $n$, and a pivot rotation at point $P$ along direction $u$ (see Figure 2). Find the rotation $R_u(\theta)$ with the smallest absolute value of $\theta$ such that the sphere no longer collides with the plane and remains above it.
Based on the problem constraints, we can construct the following system of equations: $$ \begin{cases} \| A' - A'' \| = r\,, \\[1ex] A' = R(A-P) + P\,, \\[1ex] A'' = A' - [(A' - B)\cdot n] \, n\,, \\[1ex] (A' - A'')\cdot n > 0\,,\\[1ex] \theta \to \displaystyle\min_{\|\theta\|} R_u(\theta)\,, \end{cases} $$ where $A'$ is $A$ after the application of rotation $R$, and $A''$ is $A'$ projected onto the plane defined by $B$ and $n$. From the first equation, we get $$ \| A' \|^2 + \|A''\|^2 - 2 A'\cdot A'' = r^2\,.$$ Using the third equation, we can express $|A''|^2$ and $A'\cdot A''$:
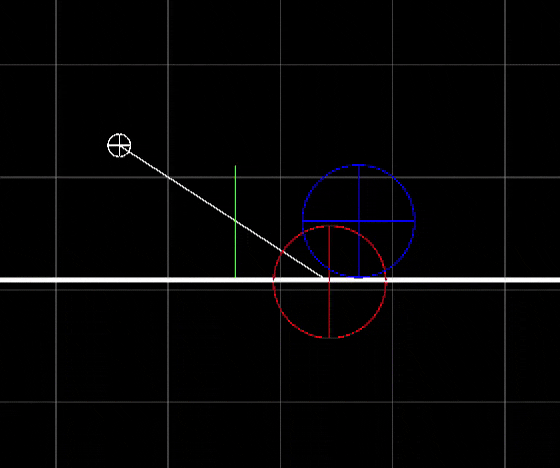
public static bool Solve(float3 A, float r, float3 B, float3 P, float3 u, float3 n, out float theta)
{
static float delta(float a, float b, float c) => a * a + b * b - c * c;
static float clip(float angle) => angle > math.PI ? angle - 2 * math.PI : angle < -math.PI ? angle + 2 * math.PI : angle;
static float2 quadratic(float a, float b, float c, float delta) => 2 * math.atan2(math.float2(b + math.sqrt(delta), b - math.sqrt(delta)), a + c);
float3 Aprim(float theta) => math.cos(theta) * (A - P) + math.sin(theta) * math.cross(u, A - P) + (1 - math.cos(theta)) * u * math.dot(u, A - P) + P;
bool accept(float theta, float minThetaAbs) => math.abs(theta) < minThetaAbs && math.dot(Aprim(theta) - B, n) > 0;
(float theta, float thetaAbs) trysolve(float a, float b, float c, float theta, float minThetaAbs)
{
var d = delta(a, b, c);
if (d >= 0)
{
var thetas = quadratic(a, b, c, d);
var theta0 = clip(thetas[0]);
var theta1 = clip(thetas[1]);
(theta, minThetaAbs) = accept(theta0, minThetaAbs) ? (theta0, math.abs(theta0)) : (theta, minThetaAbs);
(theta, minThetaAbs) = accept(theta1, minThetaAbs) ? (theta1, math.abs(theta1)) : (theta, minThetaAbs);
}
return (theta, minThetaAbs);
}
// Quick exit: if collision sphere does not collide with plane and its above of it.
var abn = math.dot(A - B, n);
if (math.abs(abn) >= r && abn > 0)
{
theta = default;
return false;
}
var a = math.dot(n, A - P) - math.dot(n, u) * math.dot(u, A - P);
var b = math.dot(n, math.cross(u, A - P));
var c = math.dot(n, B - P) - math.dot(n, u) * math.dot(u, A - P);
theta = float.NaN;
var minThetaAbs = float.MaxValue;
(theta, minThetaAbs) = trysolve(a, b, c + r, theta, minThetaAbs);
(theta, minThetaAbs) = trysolve(a, b, c - r, theta, minThetaAbs);
return !math.isnan(theta);
}